Dev Log: Areas of Effect
My most recent development in my game's crazy system is implementing an area of effect. Right now areas of effect can keep track of any Entities that enter them with a "Combatant" component. I'm using the Combatant component to keep track of anything in the scene that can either deal or receive damage in some way. Here's an area of effect that increases the player's "TimeRate" stat:
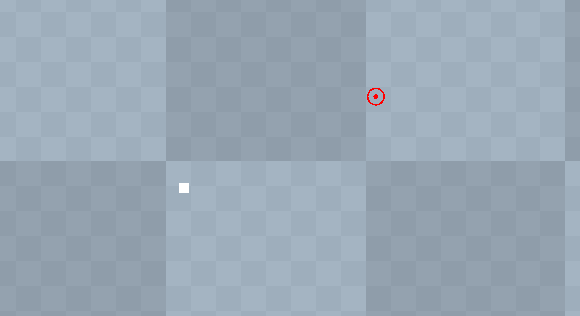
When the TimeRate stat is modified the player's weapons will fire faster, and anything else that depends on that stat is also changed. I think the way I have it set up right now any sort of regeneration status will be effected by the TimeRate, so if I had a health regeneration modifier then it would be applied faster than normal.
The way I have things set up right now is that all entities in the scene that are combatants have some sort of core stats table. These stats determine all kinds of things for each of those entities, and things like areas of effect can just apply modifiers to those stats and hopefully the entities will react accordingly. This is all totally new stuff to me and I'm learning a lot as I go, but progress is kinda slow as the more I learn about this stuff the more I know about what I could be doing better -- so I end up scrapping stuff and writing it better as I realize it.
Not quite as fast as a game jam scenario, but I don't want to code myself into a corner when it comes to making a system like this!
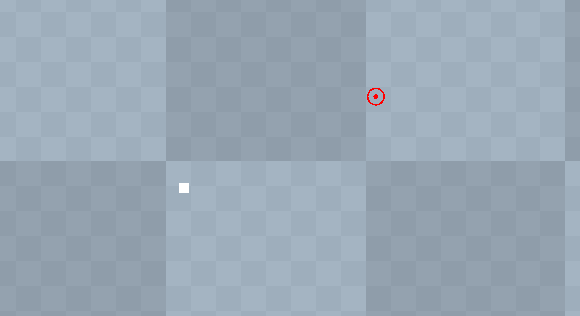
When the TimeRate stat is modified the player's weapons will fire faster, and anything else that depends on that stat is also changed. I think the way I have it set up right now any sort of regeneration status will be effected by the TimeRate, so if I had a health regeneration modifier then it would be applied faster than normal.
The way I have things set up right now is that all entities in the scene that are combatants have some sort of core stats table. These stats determine all kinds of things for each of those entities, and things like areas of effect can just apply modifiers to those stats and hopefully the entities will react accordingly. This is all totally new stuff to me and I'm learning a lot as I go, but progress is kinda slow as the more I learn about this stuff the more I know about what I could be doing better -- so I end up scrapping stuff and writing it better as I realize it.
Not quite as fast as a game jam scenario, but I don't want to code myself into a corner when it comes to making a system like this!
No Comments