Otter on Nuget
Thanks to the help of some friendly internet folks I finally got a process to get Otter up and running on Nuget! You can check out the latest main build of Otter all wrapped up as a Nuget package right here.
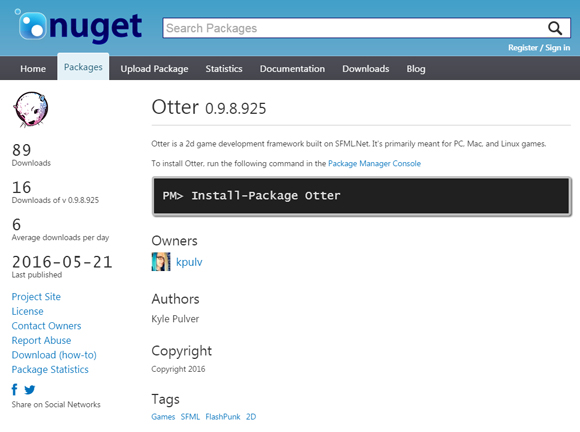
I know that it was in demand for awhile now but for the longest time I just couldn't figure out the process needed to get Otter up there. Now you can enjoy all the luxuries of the Otter game making framework without having to go download files and move them around and all that junk. Just install the package from Nuget and you're good to go!
Also somehow the name Otter wasn't taken already?
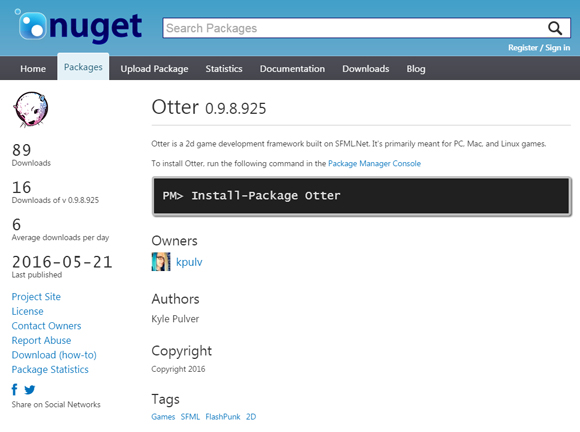
I know that it was in demand for awhile now but for the longest time I just couldn't figure out the process needed to get Otter up there. Now you can enjoy all the luxuries of the Otter game making framework without having to go download files and move them around and all that junk. Just install the package from Nuget and you're good to go!
Also somehow the name Otter wasn't taken already?
No Comments